Introduction
Artificial Intelligence หรือ AI นั้นถูกพัฒนาอย่างต่อเนื่องในหลายปีมานี้ เราสามารถนิยาม AI ได้ว่าเป็นการพัฒนาระบบอัจฉริยะที่สามารถปฏิบัติงานที่ต้องใช้สติปัญญาของมนุษย์
งานเหล่านี้รวมถึงการเรียนรู้ การใช้เหตุผล การแก้ปัญหา การตัดสินใจ และการรับรู้โดยที่ Python ถือได้ว่าเป็นหนึ่งในภาษาโปรแกรมยอดนิยมที่ใช้ในการพัฒนาระบบ AI
Python และ AI
Python เป็นภาษาโปรแกรมระดับสูงที่แปลความหมายซึ่งมีไวยากรณ์ที่เรียบง่ายและไลบรารี่ที่ใช้งานง่าย มันถูกใช้เพื่อวัตถุประสงค์ต่างๆ รวมถึงการพัฒนาเว็บ Data science และการพัฒนา AI
Python มีไลบรารีต่างๆ ที่สามารถใช้ในการพัฒนาระบบ AI ได้ อย่างเช่น TensorFlow, PyTorch และ Scikit-Learn
TensorFlow เป็น open-source machine learning library ที่พัฒนาโดย Google ใช้ในการพัฒนา Neural networks ซึ่งเป็นหัวใจสำคัญของระบบ AI ส่วนใหญ่ TensorFlow มีอินเทอร์เฟซที่ใช้งานง่ายสำหรับการพัฒนา Neural networks และมีเอกสารประกอบที่ดีเยี่ยม
PyTorch เป็น Open-source machine learning library ที่ใช้กันอย่างแพร่หลายสำหรับการพัฒนา AI ได้รับการพัฒนาโดย Facebook และเป็นที่รู้จักในด้านความยืดหยุ่นและใช้งานง่าย PyTorch นำเสนอกราฟการคำนวณแบบไดนามิกที่ทำให้ง่ายต่อการสร้างและดีบัก Neural networks
Scikit-Learn คือ Machine-learning library ที่มีอัลกอริทึมต่างๆ สำหรับการ Classification, regression และการ Clustering ใช้งานง่ายและมีเอกสารประกอบที่ยอดเยี่ยม ทำให้เป็นตัวเลือกที่เหมาะสำหรับผู้เริ่มต้นในการพัฒนา AI
AI Concepts
ก่อนที่เราจะไปพัฒนา AI ด้วย Python เราจำเป็นที่จะต้องเข้าใจคอนเซ็ปสำคัญๆ ในการพัฒนา AI กันก่อน คอนเซ็ปเหล่านี้รวมไปถึง Machine learning, deep learning, neural networks และ natural language processing.
Machine learning เป็นส่วนย่อยของ AI ที่เกี่ยวข้องกับการใช้เทคนิคสถิติเพื่อช่วยเครื่องจักรปรับปรุงประสิทธิภาพในงานที่กำหนดไว้ โดยการฝึกโมเดลการเรียนรู้ของเครื่องด้วยชุดข้อมูลและใช้โมเดลนั้นในการทำนายข้อมูลใหม่
Deep learning เป็นส่วนย่อยของ machine learning ที่ใช้ neural network เพื่อประมวลผลงานที่ซับซ้อน โดย neural network เป็นชุดของอัลกอริทึมที่ถูกจำลองตามโครงสร้างของสมองมนุษย์ และใช้ในการจำรูปแบบของข้อมูลและทำนายข้อมูลตามรูปแบบนั้นๆ
Natural language processing หรือ NLP เป็นส่วนหนึ่งของ AI ที่ใช้อัลกอริทึมในการประมวลผลและวิเคราะห์ภาษามนุษย์ โดย NLP นั้นนำไปใช้ในแอปพลิเคชันต่าง ๆ เช่น chatbot, การวิเคราะห์ความรู้สึกของผู้ใช้งาน (sentiment analysis) และการแปลภาษา
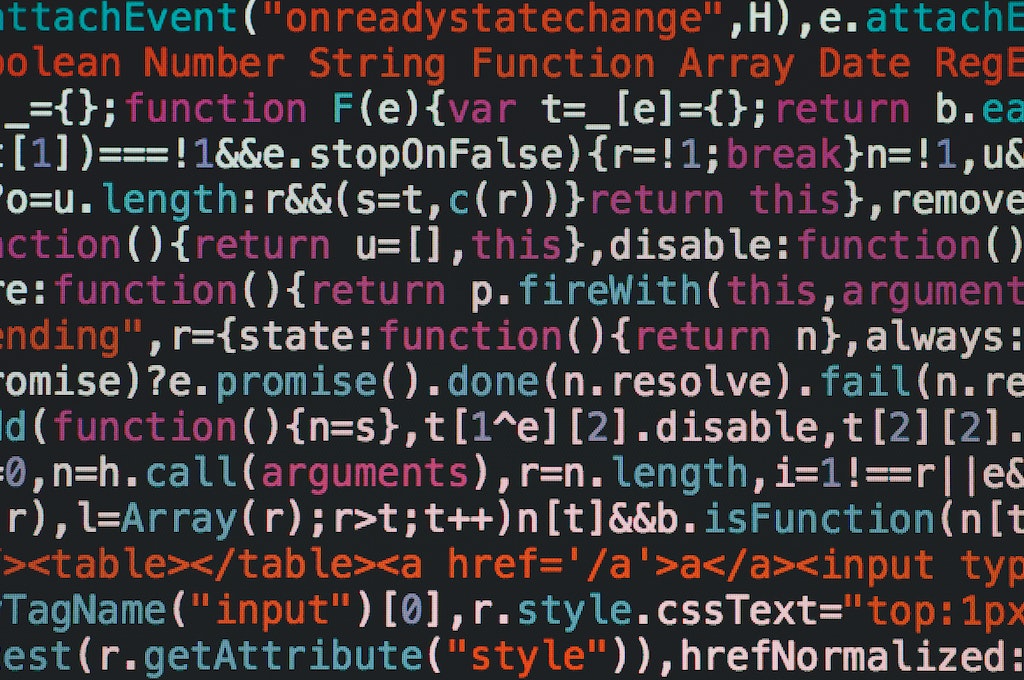
Python Code
เอาล่ะตอนนี้เราดูโค้ด Python ที่แสดงให้เห็นว่า Python สามารถใช้ในการพัฒนาระบบ AI ได้อย่างไร
Machine Learning ด้วย Scikit-Learn
โค้ดต่อไปนี้สาธิตวิธีใช้ Scikit-Learn เพื่อพัฒนาโมเดลการเรียนรู้ของเครื่องที่ทำนายราคาบ้านตามลักษณะของบ้าน
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Load the Boston Housing dataset
boston = datasets.load_boston()
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(boston.data, boston.target, test_size=0.2, random_state=42)
# Train a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Evaluate the model on the testing set
score = model.score(X_test, y_test)
print("Model Score:", score)
Deep Learning ด้วย TensorFlow
โค้ดต่อไปนี้สาธิตวิธีใช้ TensorFlow เพื่อพัฒนาโมเดล Deep Learning ที่สามารถจำตัวเลขที่เขียนด้วยมือได้
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras.layers import Dense, Flatten, Conv2D
# Load the MNIST dataset
mnist = keras.datasets.mnist
(train
# Split the data into training and testing sets
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# Normalize the data
x_train = x_train / 255.0
x_test = x_test / 255.0
# Define the model architecture
model = keras.Sequential([
Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)),
Conv2D(64, kernel_size=(3, 3), activation='relu'),
Flatten(),
Dense(10, activation='softmax')
])
# Compile the model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the model
model.fit(x_train.reshape(-1, 28, 28, 1), y_train, epochs=5, batch_size=32, validation_data=(x_test.reshape(-1, 28, 28, 1), y_test))
# Evaluate the model on the testing set
test_loss, test_acc = model.evaluate(x_test.reshape(-1, 28, 28, 1), y_test)
print('Test accuracy:', test_acc)
การประมวลภาษาธรรมชาติด้วย NLTK
โค้ดต่อไปนี้สาธิตวิธีใช้ Natural Language Toolkit (NLTK) เพื่อทำ Sentiment analysis ของข้อมูลข้อความที่มีอยู่ในชุดข้อมูล
import nltk
from nltk.sentiment.vader import SentimentIntensityAnalyzer
# Load the text dataset
with open('text_dataset.txt', 'r') as f:
text = f.read()
# Initialize the sentiment analyzer
sia = SentimentIntensityAnalyzer()
# Perform sentiment analysis on the text dataset
sentiments = []
for sentence in nltk.sent_tokenize(text):
sentiment = sia.polarity_scores(sentence)
sentiments.append(sentiment)
# Print the average sentiment score
avg_sentiment = sum([sentiment['compound'] for sentiment in sentiments]) / len(sentiments)
print('Average sentiment score:', avg_sentiment)
สรุป
Python เป็นตัวเลือกที่ดีมากๆ สำหรับการพัฒนาระบบ AI เนื่องจากความง่าย ความยืดหยุ่นของมัน และด้วยการที่มันมีหลาย Library เช่น TensorFlow, PyTorch และ Scikit-Learn Python ทำให้ง่ายต่อการพัฒนาแอปพลิเคชัน Machine learning, deep learning และ NLP applications.
การเข้าใจแนวคิดหลักของ AI เช่น Machine learning, deep learning, neural networks และ NLP นั้นเป็นสิ่งสำคัญสำหรับการพัฒนาระบบ AI ให้มีประสิทธิภาพได้ ด้วย Python และแนวคิด AI ในมือของนักพัฒนา พวกเขาสามารถสร้างแอปพลิเคชัน AI ให้เป็นนวัตกรรมที่มีศักยภาพในการเปลี่ยนแปลงโลกได้