ก็มาต่อจากพาร์ทที่แล้วที่เราพาทุกคนไปทำความเข้าใจกับพื้นฐาน Python ใน Data Science กันแล้ว คราวนี้ถึงคราวที่เราจะมาต่อกันแล้วนะครับ ในบทความนี้ เราจะกล่าวถึงไวยากรณ์ของไพธอนมากขึ้น
- Data Structures
- Logic Control Flow and Filtering
- Loops
1. Data Structures
โครงสร้างข้อมูลเป็นรูปแบบที่สามารถเก็บข้อมูลไว้ในหน่วยความจำได้ Python มีโครงสร้างข้อมูลในตัวหลายตัว ดังนั้นโปรแกรมเมอร์จึงไม่จำเป็นต้องดำเนินการเหล่านี้ตั้งแต่เริ่มต้น ซึ่งได้แก่:
- Dictionary
- Tuple
- Set
- List (กล่าวถึงในบทความก่อนหน้านี้)
1.1 Dictionaries
ใน Python พจนานุกรมคือชุดของคู่ key-value เป็นโครงสร้างข้อมูลที่ให้คุณจัดเก็บและดึงค่าโดยใช้คีย์แทนดัชนี เช่นเดียวกับที่คุณทำกับ list หรือ tuple
พจนานุกรมประกาศใช้โดยใช้เครื่องหมายปีกกา {} และสามารถเติมด้วยคู่ key-value ที่คั่นด้วยเครื่องหมายทวิภาค (:)
#storing data by using lists
pop = [30.55, 2.77, 39.21]
countries = ["afghanistan", "albania", "algeria"]
...
#storing data by using dictionaries, Dictionaries are supposed
#to be more convennient to store data about data
world = {"afghanistan":30.55,"albania":2.77, "algeria":39.21}
world["albania"]
output:
2.77
world = {"afghanistan":30.55,"albania":2.77,"algeria":39.21,"albania":2.81}
world
output:
{'afghanistan': 30.55,'albania': 2.81,'algeria': 39.21}
1.1.1 Adding data in a dictionary
#adding another country(key) and population(value) in the world dictionary
world["sealand"] = 0.000027
world
output:
{'afghanistan': 30.55,'albania': 2.81,'algeria': 39.21,'sealand': 2.7e-05}
#checking the addition of key in dictionary
"sealand" in world
output:
True
1.1.2 Deleting data from a dictionary
del(world["sealand"])
world
output:
{'afghanistan': 30.55, 'albania': 2.81, 'algeria': 39.21}
#sealand (key and value) has been deleted
1.1.3 Lists vs. Dictionary
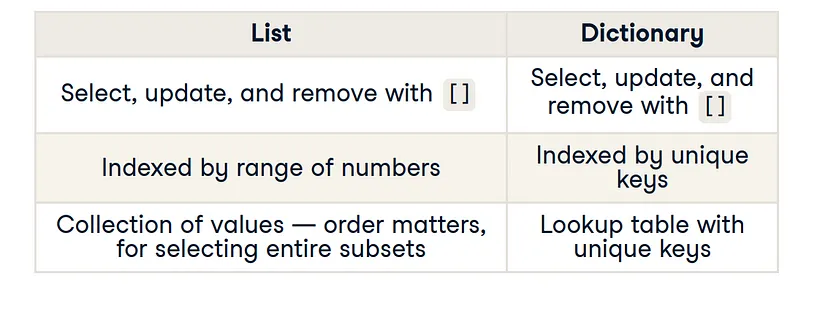
1.2 Tuples
ใน Python ทูเพิลเป็นลำดับขององค์ประกอบที่ไม่เปลี่ยนรูป ซึ่งหมายความว่าเมื่อสร้างทูเพิลแล้ว จะไม่สามารถแก้ไขเนื้อหาได้ Tuples คล้ายกับ listsใน Python แต่มีความแตกต่างที่สำคัญสองประการ:
- ทูเพิลจะอยู่ในวงเล็บ () ส่วน lists จะอยู่ในวงเล็บเหลี่ยม []
- Tuples ไม่อาจเปลี่ยนแปลงได้ในขณะที่ lists เปลี่ยนแปลงได้
# tuple are used with "round brackets"
t = (1,2,3)
# tuple indexing is just same as list indexing we covered before
t[1]
output:
2
# tuple slicing is just same as list slicing we covered before
t[1:]
output:
(2, 3)

1.3 Sets
ใน Python เซตคือชุดขององค์ประกอบที่ไม่ซ้ำแบบไม่มีลำดับ ซึ่งหมายความว่าแต่ละองค์ประกอบในชุดจะไม่ซ้ำกัน (เช่น สามารถปรากฏได้เพียงครั้งเดียวในชุด) และองค์ประกอบจะไม่ถูกจัดเก็บไว้ในลำดับใดๆ ชุดจะใช้เมื่อคุณต้องการเก็บคอลเลกชันขององค์ประกอบ แต่คุณไม่สนใจเกี่ยวกับลำดับหรือรายการที่ซ้ำกัน
set = {1,2,3}
# sets are collections of unique items
{1,2,3,1,2,1,2,3,3,3,3,2,2,2,1,1,2}
output:
{1, 2, 3}
# set item add
set.add(9)
set
output:
{1, 2, 3, 9}
# set allow intersection, difference, union among other operations
s1 = {1,2,3}
s2 = {3,4,5}
intersection = s1.intersection(s2)
print("intersection is:", intersection)
union = s1.union(s2)
print("union is:", union)
difference = s1.difference(s2)
print("difference is:", difference)
output:
intersection is: {3}
union is: {1, 2, 3, 4, 5}
difference is: {1, 2}
# Ashort hand for
a={1,3,4,6,'g'}
b = {2, 3, 4, 5}
print("Intersection: ",a & b)
print("Union: ",a | b)
output:
Intersection: {3, 4}
Union: {1, 2, 3, 4, 5, 6, 'g'}
2. Logic Control Flow and Filtering
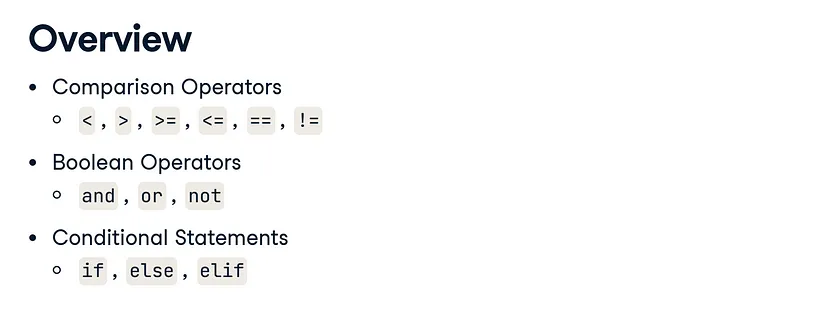
2.1 If
#basic syntax
if condition :
expression
z = 4
if z % 2 == 0 :
print("checking " + str(z))
print("z is even")
output:
checking 4
z is even
2.2 else
#basic syntax
if condition :
expression
else :
expression
z = 5
if z % 2 == 0 : # False
print("z is even")
else :
print("z is odd")
output:
z is odd
2.3 elif
#basic syntax
if condition :
expression
elif condition :
expression
else :
expression
z = 3
if z % 2 == 0 :
print("z is divisible by 2") # False
elif z % 3 == 0 :
print("z is divisible by 3") # True
else :
print("z is neither divisible by 2 nor by 3")
output:
z is divisible by 3
3. Loops
- while
- for
3.1 while
while condition :
expression
error = 50.0
# 0.78125
while error > 1: # False
error = error / 4
print(error)
output:
12.5
3.125
0.78125
3.2 for loop
#basic syntax
for var in seq :
expression
# for each var in seq, execute expression
fam = [1.73, 1.68, 1.71, 1.89]
for height in fam :
print(height)
output:
1.73
1.68
1.71
1.89
– enumerate, loops over list, dictionary, NumPy arrays
fam = [1.73, 1.68, 1.71, 1.89]
for index, height in enumerate(fam) :
print("index " + str(index) + ": " + str(height))
output:
index 0: 1.73
index 1: 1.68
index 2: 1.71
index 3: 1.89
for c in "family" :
print(c.capitalize())
output:
F
A
M
I
L
Y
#looping over dictionary
world = { "afghanistan":30.55,"albania":2.77,"algeria":39.21 }
for key, value in world.items() :
print(key + "--" + str(value))
output:
algeria -- 39.21
afghanistan -- 30.55
albania -- 2.77
import numpy as np
np_height = np.array([1.73, 1.68, 1.71, 1.89, 1.79])
np_weight = np.array([65.4, 59.2, 63.6, 88.4, 68.7])
bmi = np_weight / np_height ** 2
for val in bmi :
print(val)
output:
21.852
20.975
21.750
24.747
21.441